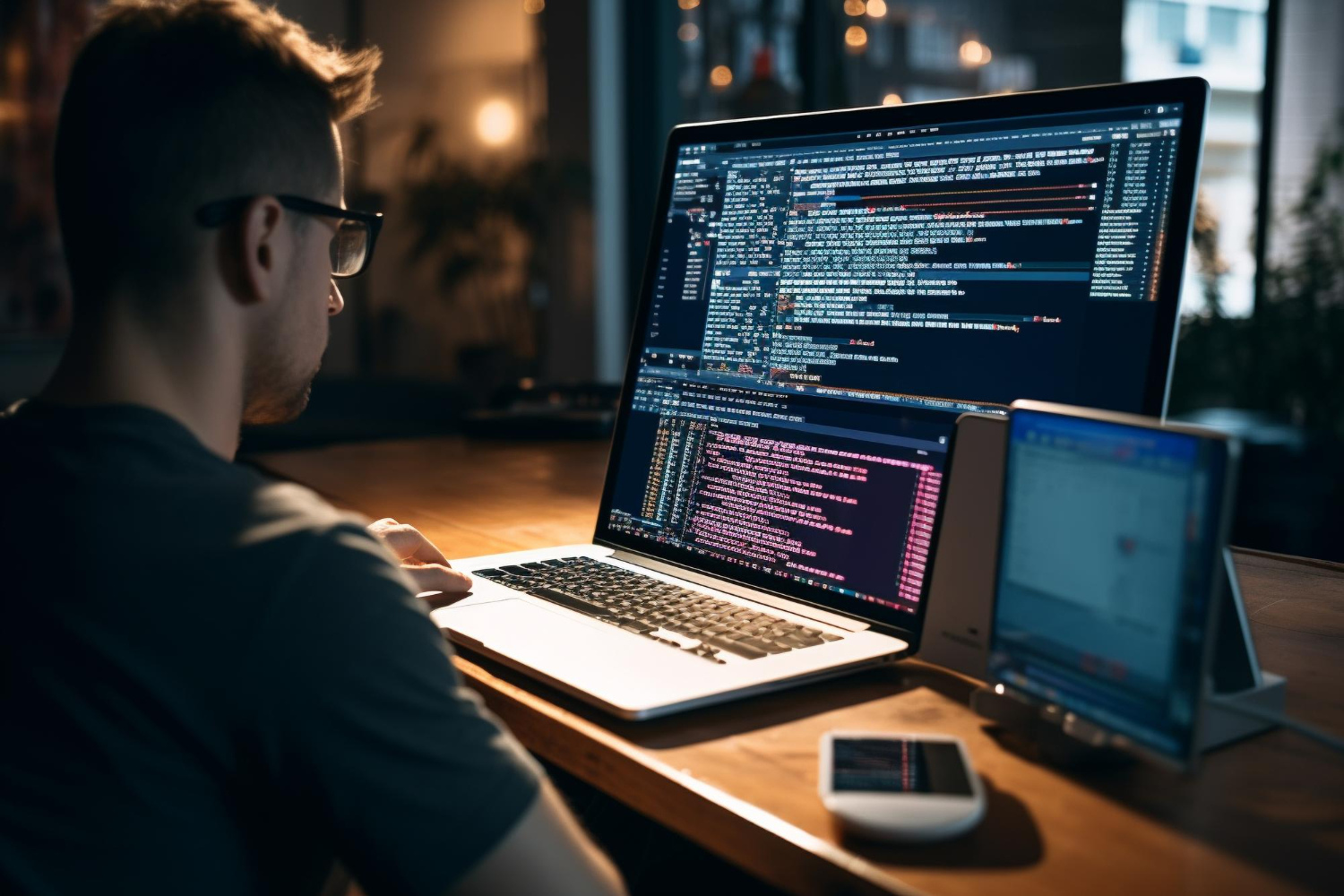
About Course
Python programming has become synonymous with versatility and efficiency in the realm of computer science. Widely recognized for its simplicity and readability, Python is a general-purpose language that finds extensive applications across various domains. From web development to data analysis, task automation to software prototyping, Python serves as a versatile tool in the arsenal of programmers, enabling them to tackle diverse challenges with ease. What sets Python apart is its accessibility; its user-friendly syntax and extensive libraries make it an ideal choice for both beginners and seasoned developers. Furthermore, Python's robust ecosystem fosters innovation, with a vibrant community continually contributing to its growth and evolution. Whether you're a web developer crafting dynamic websites, a data scientist extracting insights from complex datasets, or an automation engineer streamlining repetitive tasks, Python empowers you to turn ideas into reality efficiently and effectively.
What I will learn?
- Understand the fundamentals of Python programming language
- Learn about Python syntax, data types, and basic operations
- Master control flow structures such as loops and conditional statements
- Develop functions and modules for code organization and reusability
- Handle exceptions and perform file operations using Python
- Implement object-oriented programming concepts such as classes and inheritance
- Explore advanced topics including GUI development, database access, and network programming
- Gain proficiency in multithreading and handling JSON data in Python
- Apply regular expressions for pattern matching and text processing
- Utilize Python libraries for working with date and time, Excel files, and JSON data
Course Curriculum
Lesson Plan – Basic Concepts
Module 1: Introduction to Python (Duration - 2 hrs)
What can Python do?
Why Python?
Good to know
Python Syntax contrasted with other programming languages.
Python Install
Module 2: Beginning Python Basics (Duration - 3 hrs)
The print statement
Comments
Python Data Structures & Data Types
String Operations in Python
Simple Input & Output
Simple Output Formatting
Operators in Python
Module 3: Python Program Flow (Duration - 3 hrs)
Indentation
The If statement and its related statement (assertion)
An example with if and its related statement (explanation)
The while loop
The for loop
The range statement
Break & Continue
Assert
Examples for looping
Module 4: Functions & Modules (Duration - 3 hrs)
Create your own functions
Functions Parameters
Variable Arguments
Scope of a Function
Function Documentations
Lambda Functions & map
Exercise with functions
Create a Module
Standard Modules
Module 5: Exceptions Handling (Duration - 3 hrs)
Errors
Exception handling with try
Handling Multiple Exceptions
Writing your own Exception
Module 6: File Handling (Duration - 2 hrs)
File handling Modes
Reading Files
Writing & Appending to Files
Handling File Exceptions
The with statement
Module 7: Classes In Python (Duration - 4 hrs)
New Style Classes
Creating Classes
Instance Methods
Inheritance
Polymorphism
Exception Classes & Custom Exceptions
Module 8: Generators and iterators (Duration - 2 hrs)
Iterators
Generators
With Statement
Data Compression
Module 9: Data Structures (Duration - 2 hrs)
List Comprehensions
Nested List Comprehensions
Dictionary Comprehensions
Functions
Default Parameters
Variable Arguments
Specialized Sorts
Module 10: Collections (Duration - 2 hrs)
namedtuple()
deque
ChainMap
Counter
OrderedDict
defaultdict
UserDict
UserList
UserString
Lesson Plan – Advance Concepts
Module 11: Writing GUIs in Python (Duration - 3 hrs)
Introduction
Components and Events
An Example GUI
The root Component
Adding a Button
Entry Widgets
Text Widgets
Check buttons
Module 12: Python SQL Database Access (Duration - 4 hrs)
Introduction
Installation
DB Connection
Creating DB Table
INSERT, READ, UPDATE, DELETE operations
COMMIT & ROLLBACK operation
Handling Errors
Module 13: Network Programming (Duration - 3 hrs)
Introduction
A Daytime Server
Clients and Servers
The Client Program
The Server Program
Module 14: Date and Time (Duration - 1 hr)
sleep
Program execution time
More methods on date/time
Module 15: Some similar topics in-detailed (Duration Time - 1 hr)
Filter
Map
Reduce
Decorators
Frozen set
Collections
Module 16: Regular expression (Duration - 1 hr)
Split
Working with special characters, dates, emails
Quantifiers
Match and find all
Character sequence and substitute
Search method
Module 17: Threads ESSENTIAL (Duration - 1 hr)
Class and threads
Multi-threading
Synchronization
Treads Life cycle
Use cases
Module 18: Multithreading
Module 19: Python JSON
Module 20: Python Excel
Target Audience
- Programmers and Developers: Professionals who want to learn Python as a new programming language or enhance their existing skills to develop software applications, websites, and automation scripts.
- Data Analysts and Data Scientists: Individuals who work with data for analysis, visualization, and machine learning tasks. Python is widely used in data science and offers libraries such as NumPy, pandas, and scikit-learn for data manipulation and analysis.
- Web Developers: Those interested in building dynamic and interactive websites using frameworks like Django or Flask, which are based on Python.
- IT Professionals: Individuals responsible for system administration, network programming, or cybersecurity tasks, as Python is used for automation, scripting, and network-related tasks.
- Students and Educators: Students pursuing degrees in computer science, data science, or related fields, as well as educators teaching programming courses, can benefit from Python Programming Training to strengthen their foundational knowledge and teaching capabilities.
- Business Professionals: Professionals from non-technical backgrounds who wish to learn Python for tasks such as data analysis, report generation, or automating repetitive tasks in their work environment.
- Entrepreneurs and Start-up Founders: Those looking to develop prototypes, MVPs (Minimum Viable Products), or automate business processes using Python for their ventures.
- Anyone Interested in Programming: Individuals with a passion for learning programming languages or exploring new technologies can enroll in Python Programming Training to develop valuable skills applicable across various domains.